Next.js SEO Optimization Techniques
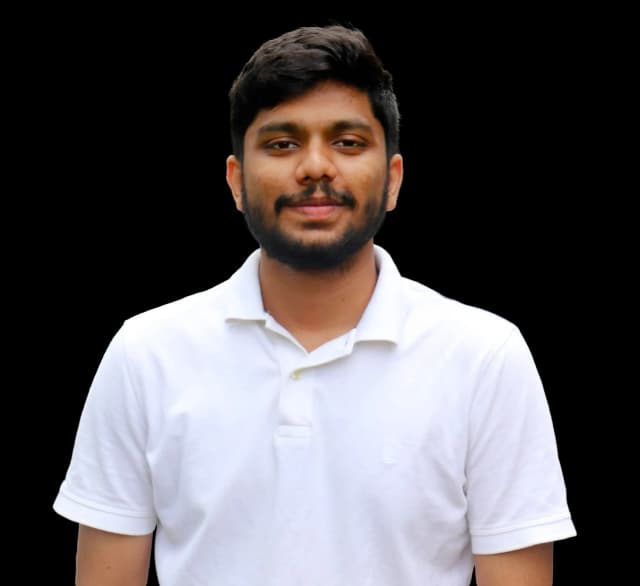
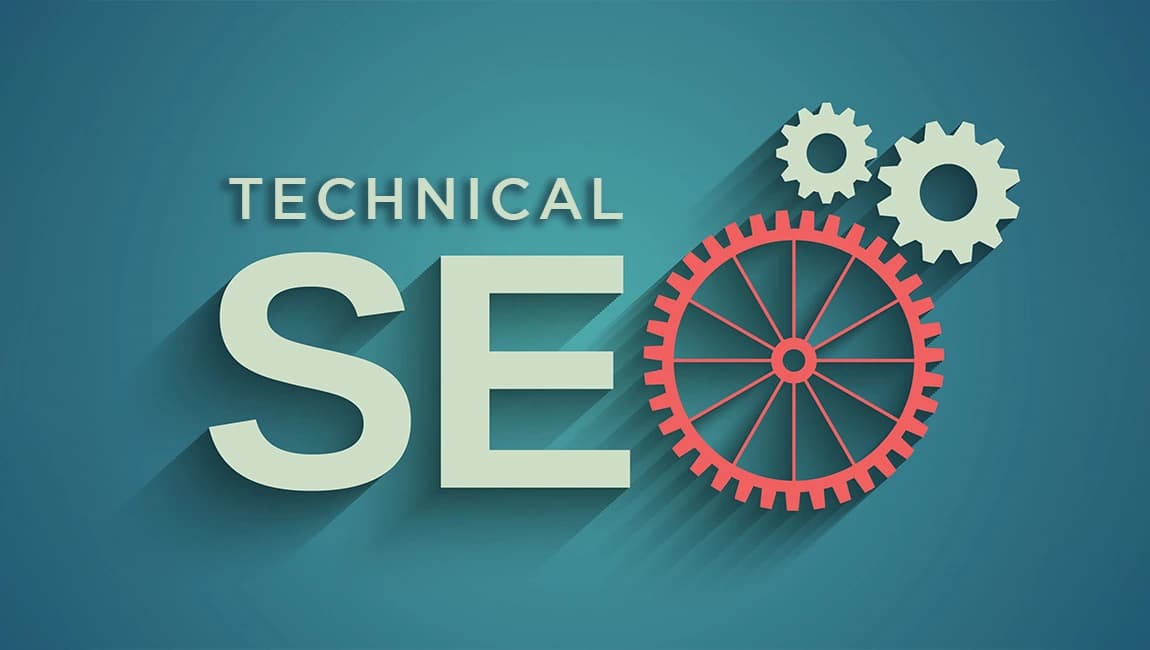
Search engine optimization (SEO) is critical for driving organic traffic to your web applications. Next.js offers tools and techniques to help you implement effective SEO strategies without compromising performance.
Understanding SEO in Next.js
SEO involves optimizing your application to ensure it ranks higher in search engine results. With server-side rendering and static generation, Next.js provides a solid foundation for achieving better SEO.
Essential SEO Features in Next.js
- •Customizable Head Tags with next/head
- •Dynamic Routing with Search-Friendly URLs
- •Optimized Performance Metrics
- •Structured Data Implementation
- •Canonical Tags for Avoiding Duplicate Content
Adding Meta Tags with next/head
import Head from 'next/head';
export default function Home() {
return (
<>
<Head>
<title>Next.js SEO Optimization</title>
<meta name="description" content="Learn effective SEO techniques for Next.js applications." />
<link rel="canonical" href="https://yourwebsite.com/nextjs-seo-optimization" />
</Head>
<main>
<h1>Welcome to Next.js SEO Optimization</h1>
</main>
</>
);
}
Structured Data in Next.js
import Head from 'next/head';
export default function Home() {
return (
<>
<Head>
<script type="application/ld+json">
{JSON.stringify({
"@context": "https://schema.org",
"@type": "WebPage",
"name": "Structured Data in Next.js",
"description": "Implementing structured data helps search engines understand your content better, improving visibility and rankings.",
"url": "https://yourwebsite.com/structured-data-nextjs"
})}
</script>
</Head>
<main>
<h1>Learn Structured Data in Next.js</h1>
</main>
</>
);
}
SEO is not just about improving rankings; it's about delivering the right content to the right audience efficiently.
By leveraging Next.js features like next/head, structured data, and performance optimizations, you can significantly improve your web application’s SEO. Coupled with a focus on user experience, these techniques ensure your application stands out in search results.